Three.jsでパーティクルを作ってみる
- 2021.07.10
- 01_技術ブログ Three.js
- #Javascript
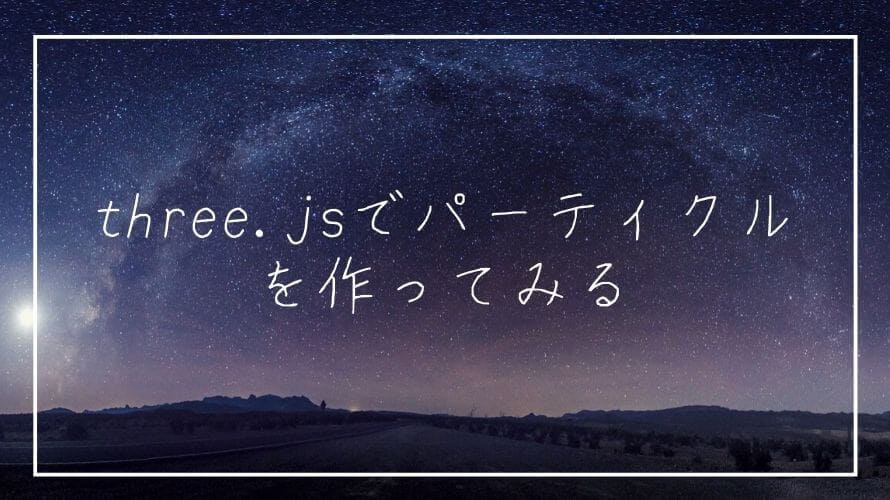
こんにちは!
プログラミング学習中のdynaです!
今回は Three.jsでパーティクルを作ってみました。
本を参考にしているので、ちょっと古いかもしれません(汗)
デモはこちらです↓※画面をマウスで動かすと回転します
See the Pen パーティクル練習1 by dyna (@sakudyna) on CodePen.
宇宙のチリくずみたい( ^ω^)・・・
プログラム作って動くと面白いですね~(^^)
では、ポイントだけ忘れないように記録に残しておきます!!
※導入の基礎の記事はこちらをご覧ください。
パーティクル作成の部分
作り方としては、
1.ジオメトリ、マテリアルを作成
2.頂点を作成してジオメトリに追加(ここが大事!)
3.メッシュを作成してシーンに追加する(表示する)
という形です。
THREE.PointsMaterial で頂点の色や見た目を変えられます。
頂点は THREE.Points で管理します。
※ジオメトリ、マテリアルって何だっけ??という方はこちらをご覧ください。
各コードで何をしているかはコメント(//部分)をご確認ください。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | //パーティクルの作成 function createParticles() { //ジオメトリ const geom = new THREE.Geometry(); //マテリアル(サイズ・頂点色彩を使うか・色) const material = new THREE.PointsMaterial({ size: 4, //サイズ transparent: true,// 透過true opacity: 0.6, //透過性の数値 vertexColors: true, //頂点色彩を使うか sizeAttenuation: true,//カメラの奥行きの減退 color: 0x3EDBF0 //色 }); //5000個のランダムな頂点作成 const range = 500; for (var i = 0; i < 5000; i++) { //THREE.Vector3(x,y,z) const particle = new THREE.Vector3( Math.random() * range - range / 2, Math.random() * range - range / 2, Math.random() * range - range / 2); //頂点をジオメトリに追加 geom.vertices.push(particle); //頂点の色を追加 const color = new THREE.Color(0xeffffc); geom.colors.push(color); } cloud = new THREE.Points(geom, material); cloud.name = "particles"; scene.add(cloud); } |
3D空間内のポイント。
3D空間での方向と長さ。
引用元:
https://threejs.org/docs/#api/en/math/Vector3
引用元:
https://threejs.org/docs/#api/en/materials/PointsMaterial
全体のコード
デモにもありますが、さらっと書き残しておきます。
※練習なので、CSSとJSは分けていません。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 | <!DOCTYPE html> <html> <head> <title>パーティクル練習</title> <script type="text/javascript"src="https://cdnjs.cloudflare.com/ajax/libs/three.js/99/three.min.js"></script> <script type="text/javascript" src="https://cdn.jsdelivr.net/npm/three@0.101.1/examples/js/controls/OrbitControls.js"></script> <style> body { margin: 0; overflow: hidden; background-color: #000000; } </style> </head> <body> <div id="WebGL-output"> </div> <script type="text/javascript"> function init() { //シーンの作成 const scene = new THREE.Scene(); //カメラの作成 const camera = new THREE.PerspectiveCamera(45, window.innerWidth / window.innerHeight, 0.1, 1000); //レンダラーの作成 const webGLRenderer = new THREE.WebGLRenderer(); webGLRenderer.setClearColor(new THREE.Color(0x000000)); webGLRenderer.setSize(window.innerWidth, window.innerHeight); //カメラの位置 camera.position.x = 0; camera.position.y = 0; camera.position.z = 150; // 滑らかにカメラコントローラーを制御する const controls = new THREE.OrbitControls(camera, document.body); controls.enableDamping = true; controls.dampingFactor = 0.2; //HTMLに書きだし document.getElementById("WebGL-output").appendChild(webGLRenderer.domElement); createParticles(); tick(); //パーティクルの作成 function createParticles() { //ジオメトリ const geom = new THREE.Geometry(); //マテリアル(サイズ・頂点色彩を使うか・色) const material = new THREE.PointsMaterial({ size: 4, //サイズ transparent: true,// 透過true opacity: 0.6, //透過性の数値 vertexColors: true, //頂点色彩を使うか sizeAttenuation: true,//カメラの奥行きの減退 color: 0x3EDBF0 //色 }); //5000個のランダムな頂点作成 const range = 500; for (var i = 0; i < 5000; i++) { //THREE.Vector3(x,y,z) const particle = new THREE.Vector3( Math.random() * range - range / 2, Math.random() * range - range / 2, Math.random() * range - range / 2); //頂点をジオメトリに追加 geom.vertices.push(particle); //頂点の色を追加 const color = new THREE.Color(0xeffffc); geom.colors.push(color); } cloud = new THREE.Points(geom, material); cloud.name = "particles"; scene.add(cloud); } // 毎フレーム時に実行されるループイベント function tick() { //cloud.rotation.x += 0.001; cloud.rotation.y += 0.0001; webGLRenderer.render(scene, camera);// レンダリング requestAnimationFrame(tick); } } window.onload = init; </script> </body> </html> |
次はテクスチャはって星空にしたいと思います(^^)*
<追記>
テクスチャの読み込みがセキリュティ的にできなそうだったので、描画してみたのがこちら。
See the Pen 星 by dyna (@sakudyna) on CodePen.
終わってしまったら、右下のReturn押してください(^^)
惑星とか入れたら宇宙旅行みたいになるかな??
ここまで読んで下さりありがとうございます。
<あわせて読みたい>
<three.jsのおすすめ学習本>